Quarkus vs. Spring Boot
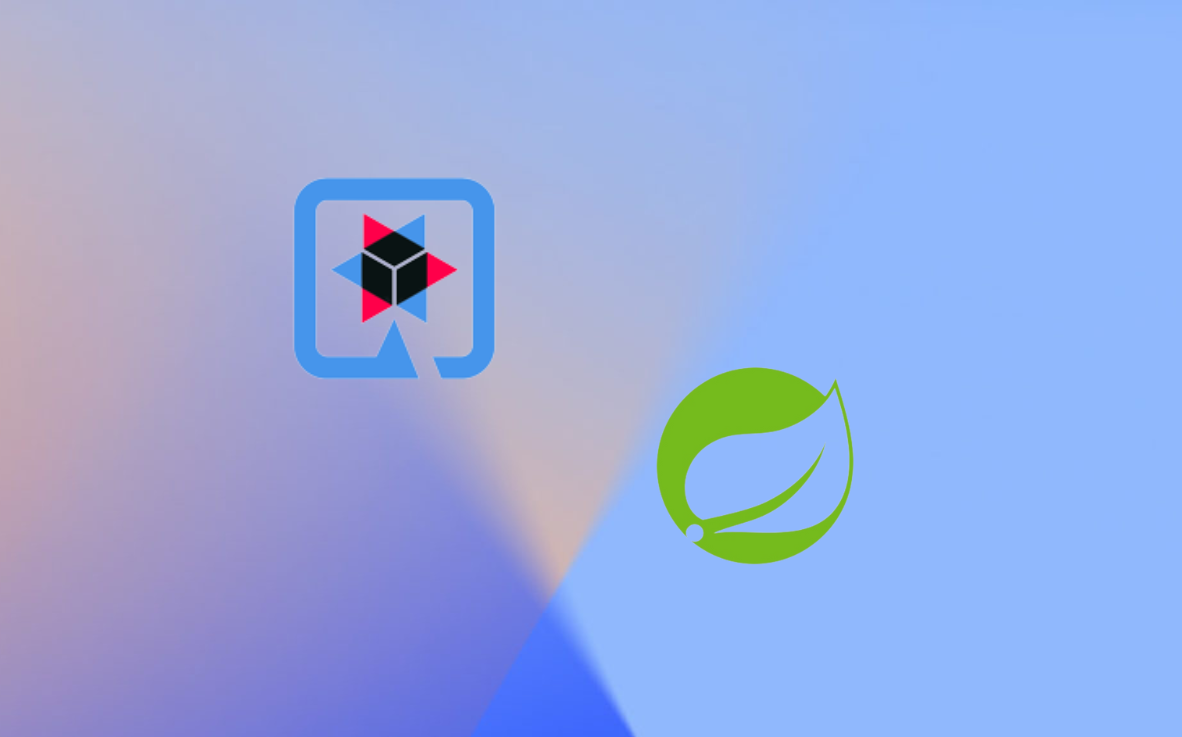
In modern application development and architecture, there has been a big push from monolithic, large applications that can do everything a product would need, to many smaller services that have a specific purpose. This onset has brought on the age of microservice frameworks (micro-frameworks), with the goal of making it easier to prototype, build, and design applications in this paradigm. Both Quarkus and Spring are big contenders vying for the spotlight when it comes to accomplishing your goals as a microservice application developer, but which should you pick?
Quarkus is a Kubernetes-Native Java framework tailored for GraalVM and HotSpot, crafted from best-of-breed Java libraries and standards. The goal of Quarkus is to make Java a leading platform in Kubernetes and serverless environments. It also offers developers a unified reactive and imperative programming model to optimally address a wider range of distributed application architectures.
Some notable features of Quarkus include:
Spring Boot is an open-source Java-based framework used to create a micro-service. It was developed by the Pivotal Team and is used to build stand-alone and production-ready spring applications. It’s a commonly chosen application framework for a MicroServices architecture.
Here are some notable features of Spring Boot:
LogicMonitor’s Metric Pipeline (where we built out a proof-of-concept of Quarkus in our environment) is deployed on the following technology stack:
Our legacy pipeline was based on Spring and Tomcat. There were a couple of things about this framework choice that we didn’t like when we inherited its maintenance and deployment, including:
In addition to Quarkus, we explored two other Java Micro-Frameworks: Helidon.io and MicroNaut.
Micronaut was probably the most comparable with Quarkus. The declarative nature of the framework was very similar and it had out of the box support for technologies we use heavily here:
The main reason we didn’t end up with Micronaut was that it was not based on the eclipse microprofile framework, but something homegrown. We felt that since Quarkus was backed by Redhat and was based on microprofile, it had a better situation regarding community support and documentation
Helidon was also one of the top contenders. It’s API framework was based on JAX-RS, and in general, we felt that it still required way too much boilerplate to get things up and running. It had a lot of similar technologies supported but it wasn’t as well integrated.
When we set out to build a POC of one of the applications in the pipeline, we set up an internal document laying out the success criteria:
APIs were very easy to design and implement. We went with Quarkus’ rest-easy implementation and found it to be both RFC compliant and similar to the JERSEY framework we were already familiar with in other applications in our infrastructure. We weren’t able to use their built-in authentication implementation, however, due to some backward compatibility issues with how the existing application authenticated requests (based on a homegrown request header token).
One of the things we really liked about the Quarkus framework was how easy it was to set up the Kubernetes Liveness/Readiness probes. All we had to do was add a dependency (smallrye-health), enable a configuration, and we had a kafka liveness/readiness probe ready to go. Adding our own health check to make sure our application was configured correctly was as easy as annotating a class, and implementing a simple interface. Then, probes were available at the “/health” (gets both liveness and readiness check results), “health/live”, and “health/ready” endpoints.
In addition to the probes, we also really liked the CDI bean injection. We found this to be more easily conceptualized than Spring’s DI implementation, and it enabled us to build a lot quicker than normal. One thing to note though, is that in order to expose metrics, those metrics must be exposed from a class with a bean-defining annotation. This is part of how microprofile works, more on metrics later.
In terms of configuration, Quarkus has support for profiles and configuration from multiple sources. At LogicMonitor, we use a central configuration service, so we ended up setting System Properties based on an eagerly-instantiated bean which polled that service for its configuration settings. We didn’t have a huge use case for the @ConfigProperty annotation because we want most of our configurations to be easily changed at runtime, and those are usually defined on a bean’s instance variables. Most of the beans in our POC app were @ApplicationScoped, so that would have required the config values to be static.
Quarkus has support for Kafka through the SmallRye Reactive Messaging framework. We had a good experience with this framework for our Kafka Producer, but not our Kafka Consumer application. The Kafka Producer is fairly easy to set up for imperative usage in this guide. There are a couple of things to be careful of with here regarding default configuration values of the producer that caused a bunch of issues for us (and a few hours of troubleshooting headache):
When it came to the Kafka Consumer we also ran into a fair bit of trouble in two main areas:
Quarkus played very nicely with our existing Java monitoring via JMX (all the garbage collection, heap, threads, uptime DataSources applied, and gathered data automatically). It also allowed us to define our own application metrics easily. In my opinion, this was one of the biggest benefits of the app: using microprofile metrics. We were able to both time and count operations through a simple annotation, that instantly makes those metrics available from a “/metrics/application” endpoint:
The @Timed Annotation gives you tons of information: min, max, mean, throughput, percentiles). This screenshot also shows the fault tolerancy feature too (which exposes its own metrics by the way). That along with the scheduled tasks was a nice treat to discover (sidenote: we discovered that the initial delay of the scheduler was not configurable, so we put in a feature request, and it was fixed within two weeks!). Okay, back to metrics:
Gauges and Counters, super easy too:
Logging in Quarkus is done via JBoss, and once we added a logging.properties file we were able to tune things no problem.
At the end of our POC and deployment across our infrastructure, we ended up with both explicit and implicit improvements from resource utilization to ease of programming in the framework. So far, we are sticking with Quarkus!
LogicMonitor is the only fully automated, cloud-based infrastructure monitoring platform for enterprise IT and managed service providers. Gain full-stack visibility for networks, cloud, servers, and more within one unified view.
Blogs
See only what you need, right when you need it. Immediate actionable alerts with our dynamic topology and out-of-the-box AIOps capabilities.