A JSON Web Token (JWT) is a URL-safe method of transferring claims between two parties as a JSON object, the data structure used to represent data in a human-readable and machine-parseable format called JavaScript Object Notation (JSON). As JWTs gain popularity with frameworks like OAuth 2.0 and standards like OpenID Connect, they become a more trusted solution for secure data exchange.
JWTs include three components: header, payload, and signature. An encrypted or signed token with a message authentication code (MAC) is known as a JSON web signature (JWS), while an encrypted token is a JWE or JSON web encryption.
For the sake of verbal communication, many developers have taken to pronouncing JWT as “jot” or “jawt”. Continuing that theme, I propose the pronunciation of JWS as “jaws” and JWE as “jawa.”
The JWT is formed by concatenating the header JSON and the payload JSON with a “.” and optionally appending the signature. The whole string is then base64 URL encoded.
This article covers the basics of JWT tokens, offers examples of JSON Web Token usage, and answers questions about best practices.
Key takeaways
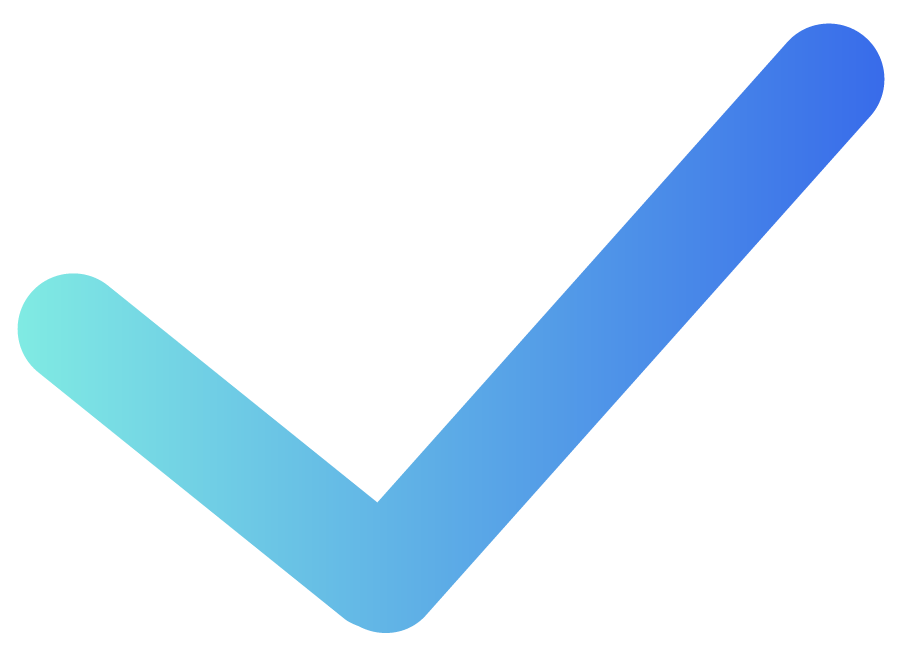
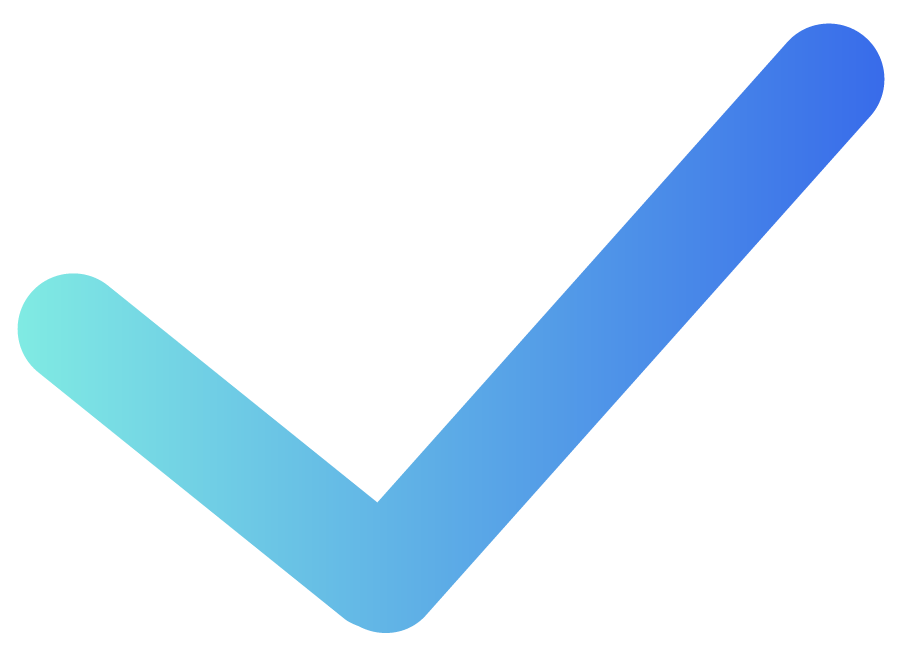
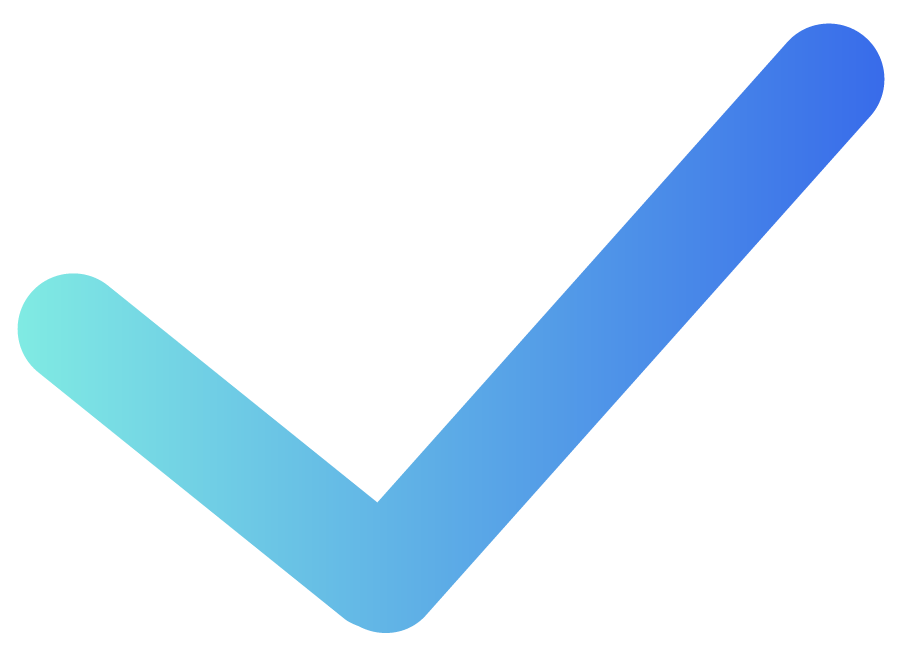
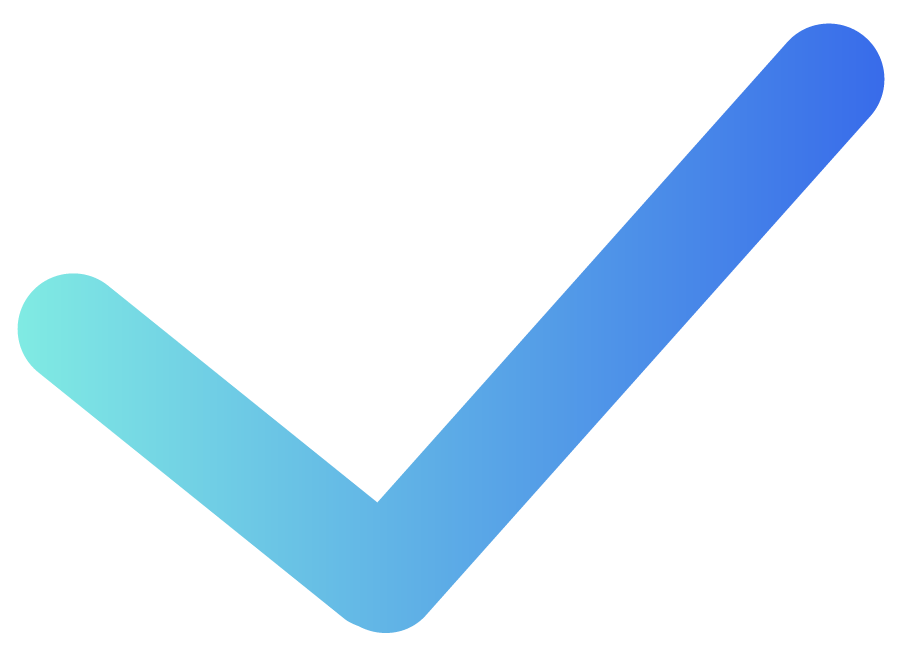
Table of contents:
- What are JSON Web Tokens?
- Example of JSON Web Tokens
- When to use JWT
- Is JWT secure?
- Best practices when implementing JWTs
- JWT Tokens vs. session tokens
- Use cases for JSON Web Tokens
- Conclusion
- FAQs about JSON Web Tokens
Example of JSON Web Tokens
JWT Example
eyJ0eXAiOiJKV1QiLCJhbGciOiJIUzI1NiJ9.eyJpc3MiOiJMTSIsImlhdCI6MTYxOTQ3MDY4MiwiZXhwIjoxNjE5NDcxODg2LCJhdWQiOiJsb2dpY21vbml0b3IuY29tIiwic3ViIjoiTmVkIn0.mqWsk4fUZ5WAPYoY9bJHI7gD8Zwdtg9DUoCll-jXCMg
Decoded Header
{
"typ": "JWT",
"alg": "HS256"
}
Decoded Payload
{
"iss": "LM",
"iat": 1619470682,
"exp": 1619471886,
"aud": "logicmonitor.com",
"sub": "Ned"
}
Signature
mqWsk4fUZ5WAPYoY9bJHI7gD8Zwdtg9DUoCll-jXCMg
When to use JWT tokens
JWTs offer practical ways for maintaining HTTP request states in stateless authentication situations. Common uses include RESTful APIs and Single Sign-On (SSO) authentication. A JWT provides state information to servers for each request. This is especially helpful for secured RESTful web services that require client authentication and authorization control.
When not to use a JWT: Do not use a JWT when a session token will do. Session tokens are a well-established method of managing client access, and their potential to introduce vulnerabilities is low.
LogicMonitor’s article about Adding and Managing Web Services provides insights on integrating JWTs with web services.
“JWTs provide a secure, stateless way to authenticate users, transforming data exchange between clients and servers.”
Security considerations for JWTs
The most common vulnerabilities of a JWT or JWS are created during implementation. As JWS does not inherently provide security, proper implementation combined with secure server monitoring with tools like Silver Peak is key to avoiding security pitfalls like the following:
- Algorithm confusion: Avoid allowing JWT headers to dictate validation algorithms, which can lead to vulnerabilities if an attacker sets the “alg” header to “none.”
- Weak secrets: Use strong, complex secrets to prevent token forgery.
- Token sidejacking: Prevent sidejacking by incorporating user context and expiration times and securely storing tokens.
Real-life comparison of encryption algorithms: HMAC vs. RSA
HMAC (hash-based message authentication code) uses a shared secret key to form and validate a signature. RSA, an asymmetric algorithm, uses a private key to form a signature and a public key to validate the digital signature.
A system using the RSA algorithm would sign with the private key and validate with the public key. If a JWT is signed using an HMAC algorithm and a public RSA key as input, then the receiving system could be fooled into using the public RSA key it knows to validate the signature using the HMAC algorithm.
This attack works when a receiving system allows a JWT header to drive token authentication. In this case, the attacker declared the public key of the asymmetric scheme to be the shared secret key of the symmetric signature scheme.
Best practices for implementing JWT tokens
To maximize the security and effectiveness of JWTs, these best practices should be followed during implementation.
- Proper authentication control: Don’t allow JWT headers to drive token authentication; use a predefined list of allowed algorithms.
- Token secrets: Avoid weak token secrets that allow attackers to generate valid tokens.
- User context: To prevent token sidejacking, add user context to tokens; random strings are provided during client authentication and kept in hardened cookies.
- Secure storage: Use hardened cookies to ensure secure storage.
- Expiration times: Set reasonable limitations on token validation dates and consider other methods for revocation for tokens that need to remain valid for extended periods.
- Scope of authorization: Issue JWTs with narrow scopes of access to secured resources.
- Require claims: Enforce limits on access points so that claims made beyond the scope limit will be rejected regardless of signature validity.
- Privileged data: Never store privileged data in a JWT or JWS, which do not hide stored data in headers or payloads.
JWT tokens vs. session tokens
JWT differs from traditional session tokens in several ways:
Stateless
JWTs are stateless and don’t require server-side storage. All information is stored within the token itself, eliminating the need for server storage and reducing web app requirements. To manage states, session tokens require server storage, so scaling these applications becomes more difficult.
Decentralization
JWTs allow for decentralized authentication. Once a user is issued a JWT, any service with the correct secret key can validate the token without contacting a central server. With session tokens, the user typically must validate their credentials with the stored session data on the server.
Flexibility
JWT can carry more information than session tokens. It can include user roles, permissions, token information, and metadata. Session data typically only contains an identifier, with the rest stored on the server.
Security
JWTs require different security considerations because sensitive data is stored on user machines. It requires encryption, proper signing, and proper handling of secret keys. Much of a session token’s security relies on server data management.
Performance
JWTs can improve web application performance by reducing server operations. For example, they may reduce the number of database lookups, improving the performance of high-bandwidth applications. Session tokens may require lookups for each request, which may make scaling more complicated.
Use cases for JSON Web Tokens
JWTs are versatile and applicable in various scenarios, such as:
Microservices
JWTs can facilitate communication between microservices. Since JWTs can authenticate with any server with secret keys, they help decentralize applications and ensure secure inter-service communication. They also help carry user context between servers, maintaining a consistent user state without a shared database.
Mobile applications
JWTs can help manage user authentication and sessions in mobile apps. This is especially important for apps that require persistent authentication in areas with intermittent connectivity. JWTs are stored securely on a device, allowing some level of offline access with cached resources while maintaining user information, permissions, and other information.
APIs and web applications
JWTs make building APIs and web applications simpler by enabling stateless authorization and rich user information. They are useful for authentication API access without server-side sessions. They simplify authentication by directly including user roles and permissions in the token, as you can see with the Zoom application authentication.
“JWTs are essential for modern applications, enabling secure, efficient communication across platforms.”
Conclusion
JWTs provide a lightweight method for managing and securing authentication and data exchange. JWT might be a good fit for organizations that require specific security measures and scalable resources.
Visit LogicMonitor’s support section or join LM’s community discussions to learn more about JWT implementation and security best practices.
FAQs about JSON Web Tokens
What is the role of JWTs in microservices architectures?
JWTs enable secure and efficient communication between microservices by allowing stateless authentication and authorization.
How can JWTs enhance mobile application security?
JWTs allow mobile apps to authenticate users securely by carrying encrypted user data and permissions, reducing the need for server-side session storage and improving performance.
What are the potential security pitfalls of JWT implementations?
Common pitfalls include algorithm confusion, weak secrets, insecure client-side storage, and improper token validation, all of which can compromise security if not addressed.
How do JWTs differ from other token types like OAuth?
JWT is a token format, while OAuth is an authorization framework. JWTs are often used as tokens within OAuth implementations.
What are the best practices for managing JWT expiration and renewal?
Implement token refresh mechanisms and set appropriate expiration times, such as short-lived access tokens and long-lived refresh tokens, to obtain new access tokens, ensuring continuous access while maintaining security.
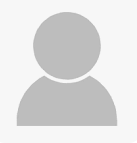
Subscribe to our blog
Get articles like this delivered straight to your inbox