The integration of Azure’s event-driven model with LogicMonitor’s monitoring capabilities offers businesses a robust solution for real-time IT infrastructure monitoring. LogicMonitor’s cloud-based platform provides a comprehensive overview of an organization’s IT infrastructure, both on cloud and on-prem. It is designed to monitor a wide range of resources, including cloud and container resources, servers, networks, storage, and applications, and every critical telemetry signal from these resources. A key feature of the LogicMonitor platform is a powerful alerting engine that can be configured to notify users of critical events across their IT infrastructure.
In this article, you will explore how to leverage LogicMonitor’s logs product, LM Logs, and the alerting engine to monitor Azure resource events. By routing Azure resource events to LM Logs, businesses can achieve an even more comprehensive and real-time overview of their Azure infrastructure.
You will use Azure Event Grid and Azure Functions to develop this integration to ensure a scalable and streamlined data flow, enabling timely responses to critical events.
What are Azure Resource Events?
Azure resource events are specific occurrences related to Azure resources that signify state changes or operations. These events are generated when resources in Azure undergo modifications, such as when a virtual machine is started, a database is updated, or a storage blob is deleted. Azure resource events are integral to Azure’s event-driven architecture, allowing developers and IT professionals to monitor, react to, and automate responses to these events. By capturing and responding to Azure resource events, organizations can enhance operational efficiency, improve security through real-time alerts, and create dynamic, responsive cloud environments. Azure Event Grid, a key service within Azure, is specifically designed to handle and route these resource events to various endpoints or applications for further processing.
What is Azure Event Grid?
Azure Event Grid is a managed event routing service integral to Azure’s serverless infrastructure. Its primary functions include:
- Event Aggregation: Event Grid consolidates all events and routes them from any source to any destination both within and outside Azure.
- Efficiency: Event Grid eliminates the need for polling, reducing associated costs and latency.
- Decoupling: Event Grid uses the publisher/subscriber model to decouple event publishers and subscribers.
- Scalability: Event Grid is designed to handle a vast volume of events, and it can be scaled out to process millions of events per second without any additional configuration.
- Flexibility: Event Grid supports both built-in and custom events, catering to a wide range of applications.
Azure Event Grid can accept events from a variety of event sources, which can be configured to trigger specific events. These events can then be handled by various Azure services like Azure Functions, Azure Logic Apps, and Azure Event Hubs. Although Azure Event Grid can accept events from any source, it is primarily used to handle events generated by Azure resources. These events are published to Azure Event Grid by Azure services and can be routed to various endpoints or applications for further processing. For a complete list of supported Azure event sources and handlers, refer to the Azure documentation on event sources and event handlers.
Using System Topics to Send Events to LM Logs
System topics are a core component of Azure Event Grid. They represent events published by specific Azure services, such as Azure Storage and Azure Container Registry. When an event, like a blob upload, occurs in the storage account, the Azure Storage service publishes this event to the system topic in Event Grid. Subsequently, the event is forwarded to the topic’s subscribers. It’s important to note that only Azure services have the privilege to publish events to system topics, ensuring a standardized and secure flow of information. In addition, Azure supports system topics across a range of services, including Azure Blob Storage, Azure Event Hubs, and Azure Kubernetes Service, among others.
Following is the high-level architecture of the integration you will build in this tutorial.


The integration of Azure resources with LogicMonitor logs is a two-step process:
- Create an Azure Function: AzureEventBridge to route events from Azure Event Grid to LogicMonitor logs.
- Configuring Azure Storage (or another resource) to publish events to Azure Event Grid.
Here is a summary of the illustrated process. You will configure the desired Azure resources to send events to an Azure Event Grid System Topic. Through an Azure Function subscription to the Event Grid topic, the Azure Function app will parse the incoming event data, transform it into a format suitable for LogicMonitor logs, and send it to LogicMonitor logs using the LogicMonitor API, thus acting as a bridge between Azure Event Grid and LogicMonitor Logs.
Step 1: Create Azure Function: AzureEventBridge
Please follow the instructions in the Azure Function documentation to create an Azure Function named AzureEventBridge using the C# script programming language. If you prefer, you can use an IDE such as Visual Studio to create the Azure Function as well by following the instructions in the Azure Function documentation for VS Code. While creating the Function app, please remember to select Azure Event Grid as the trigger type for the Azure Function. Once you have created the Function app, you can use the following code to implement the logic.
#r "Azure.Messaging.EventGrid"
#r "System.Text.Json"
#r "System.Net.Http.Json"
using Azure.Messaging.EventGrid;
using System.Globalization;
using System.Net.Http.Json;
using System.Text.Json;
using System.Text.Json.Serialization;
public static async Task Run(EventGridEvent eventGridEvent, ILogger log)
{
using (var httpClient = new HttpClient())
{
// Prepare the HTTP request to send data to LogicMonitor
httpClient.DefaultRequestHeaders.Clear();
var lmBearerToken = System.Environment.GetEnvironmentVariable("LMBearerToken", EnvironmentVariableTarget.Process);
httpClient.DefaultRequestHeaders.Authorization = new System.Net.Http.Headers.AuthenticationHeaderValue("Bearer", lmBearerToken);
httpClient.DefaultRequestHeaders.UserAgent.Add(new System.Net.Http.Headers.ProductInfoHeaderValue("AzEventBridgeFx", "1.0.0"));
var payload = new Payload[]
{
new Payload(){
// Payload attributes are indexed by LogicMonitor and can be used to create custom alert rules
Message = JsonSerializer.Serialize(eventGridEvent),
EventTime = eventGridEvent.EventTime.ToString("o", CultureInfo.InvariantCulture),
EventType = eventGridEvent.EventType,
Topic = eventGridEvent.Topic,
Subject = eventGridEvent.Subject
}
};
var response = await httpClient.PostAsJsonAsync("https://<your-account-name>.logicmonitor.com/rest/log/ingest", payload);
response.EnsureSuccessStatusCode();
}
}
// LogicMonitor requires the payload to be in a specific format. You can add additional attributes to the payload as needed.
public class Payload
{
public string Message { get; set; }
public string EventTime { get; set; }
public string EventType { get; set; }
public string Topic { get; set; }
public string Subject { get; set; }
}
The Function uses the LogicMonitor Logs Ingestion API and a simple Bearer authentication to send the event data to LogicMonitor logs. To generate a bearer token for your application, please follow the instructions in the LogicMonitor documentation and add the bearer token as an Application setting named LMBearerToken to the Azure Function, as shown in the following screenshot.
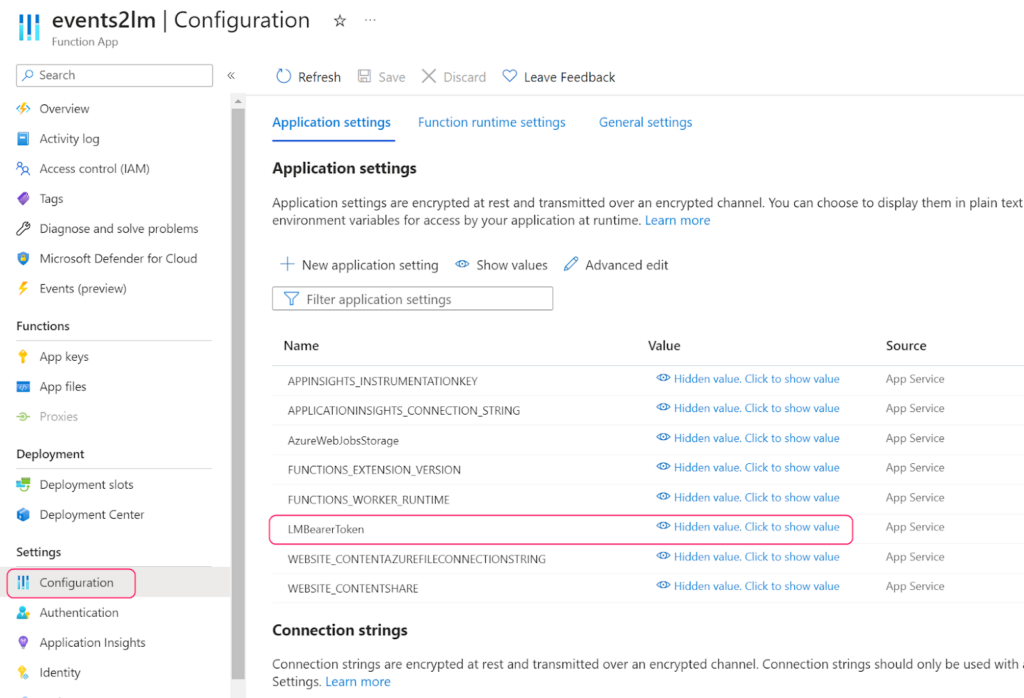
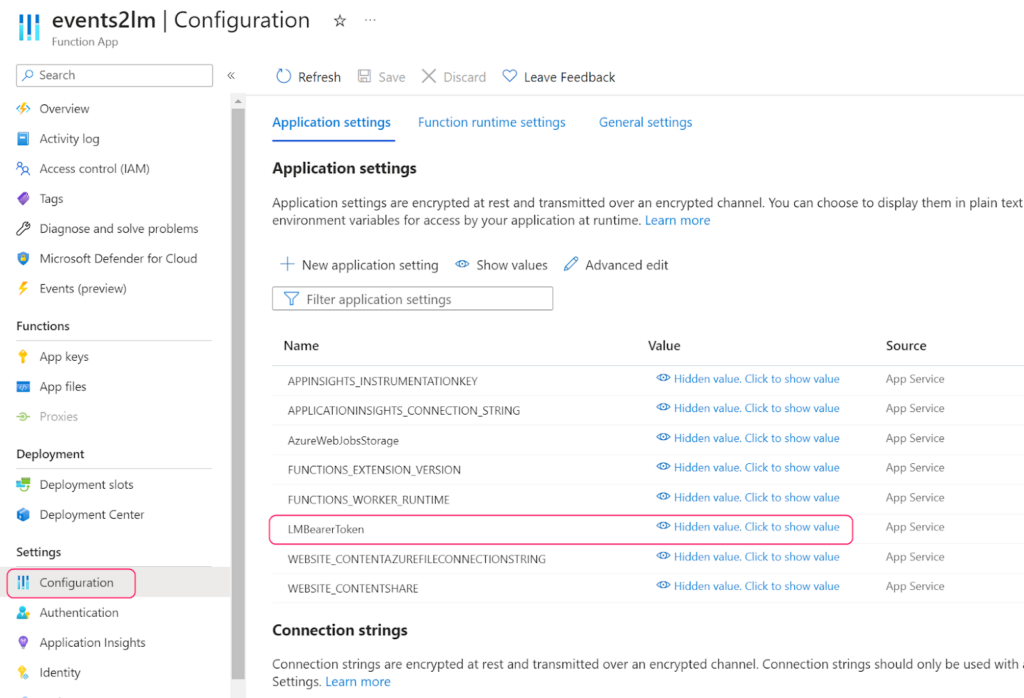
You will now configure Azure Storage to publish events to Azure Event Grid and configure Azure Event Grid to forward these events to the AzureEventBridge function.
Step 2: Configure Azure Storage to Publish Events to Azure Event Grid
Please follow the Azure learn guide to configure Azure Storage to publish events to Azure Event Grid. Once you have configured Azure Storage to publish events to Azure Event Grid, you will need to configure Azure Event Grid to forward these events to the AzureEventBridge function.
The following video shows how you can build the integration with just a few clicks in the Azure portal:
With the integration in place, you can now set up alert conditions in LogicMonitor logs to ensure that you are notified of critical events such as blob deletion.
Set Up Alert Conditions in LM Logs
Perform a few test operations on your Azure Storage account to generate some events. You can immediately see the events in LogicMonitor logs as shown in the following screenshot:
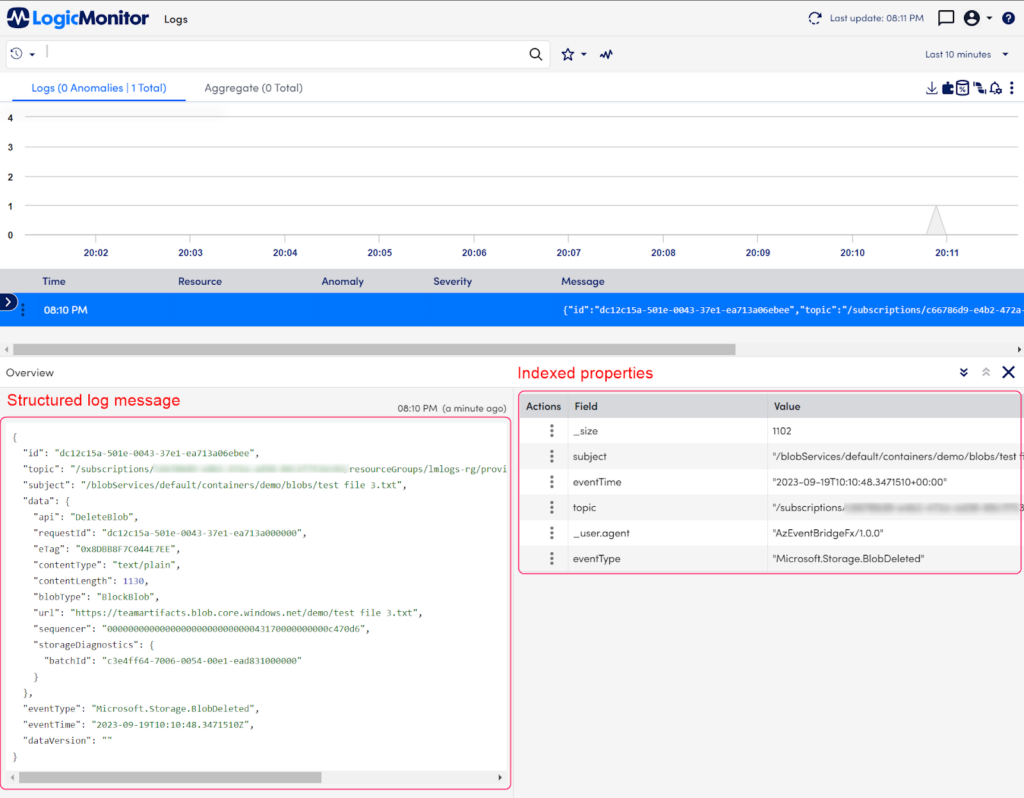
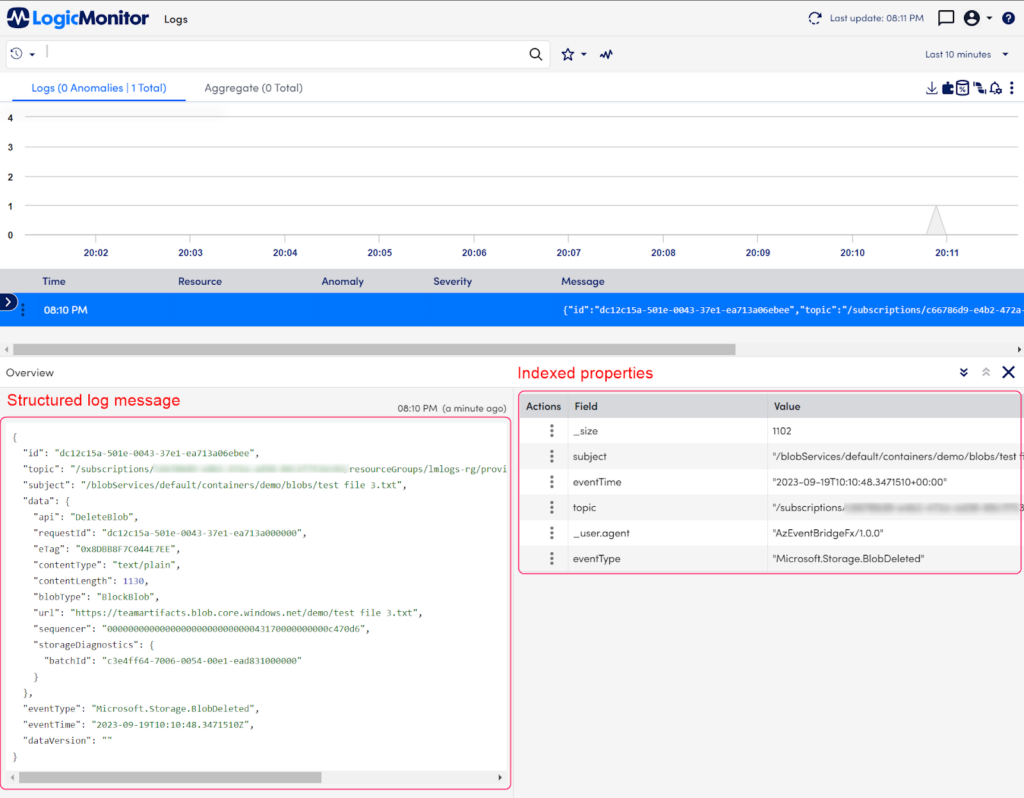
To ensure that you are notified of critical events, set up alert conditions in LogicMonitor logs to notify users when a blob is deleted from Azure Blob Storage. To do this, you will need to create a new alert rule in LogicMonitor logs as follows:
First, create a new LM Logs pipeline using the following logs query:
_lm_user_agent = "AzEventBridgeFx/1.0.0."
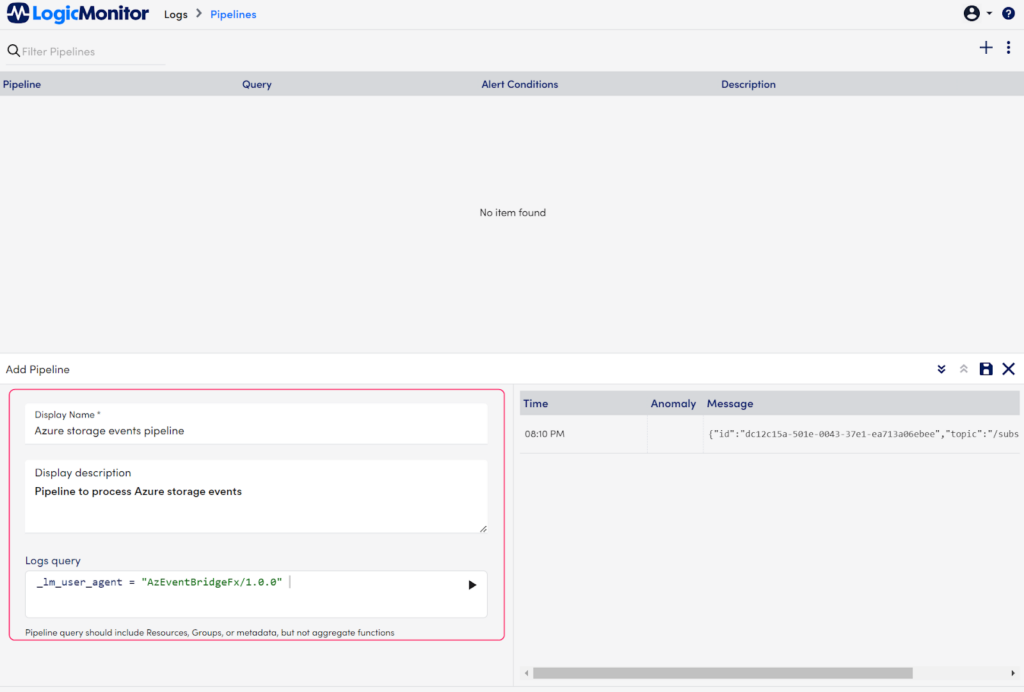
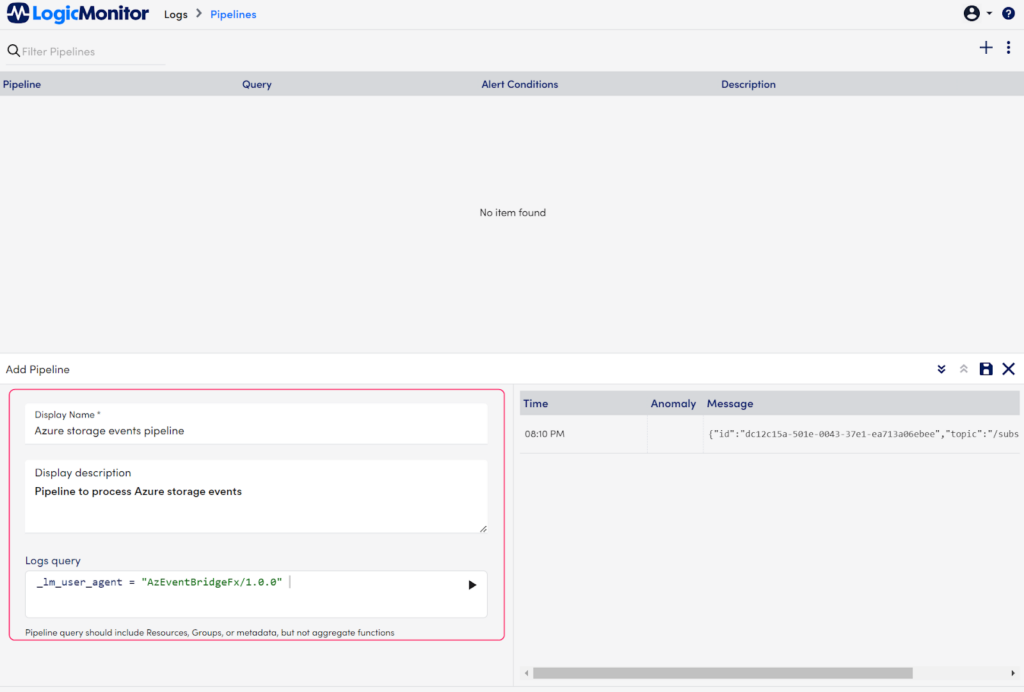
Next, create a new alert rule in the pipeline with the following filter query:
eventType = "Microsoft.Storage.BlobDeleted"
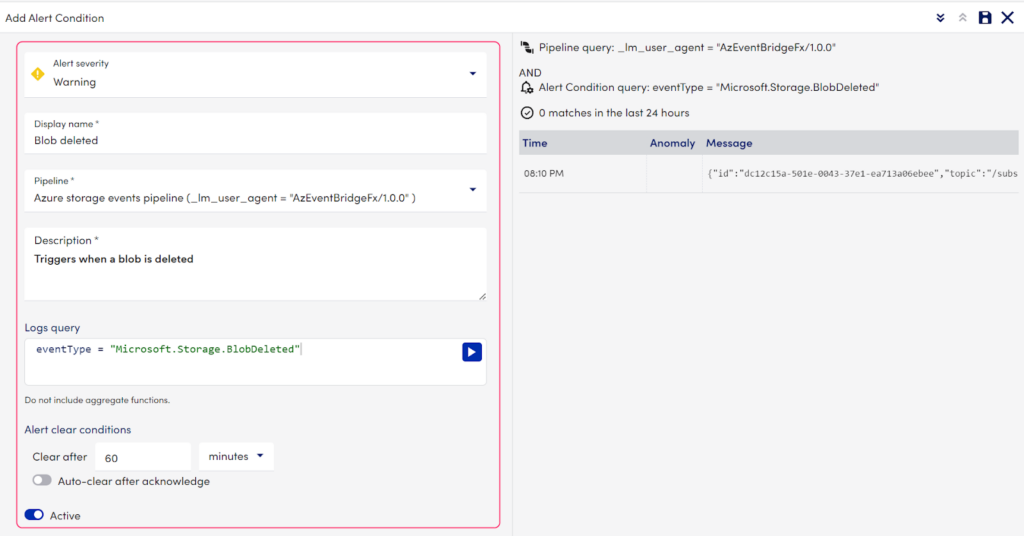
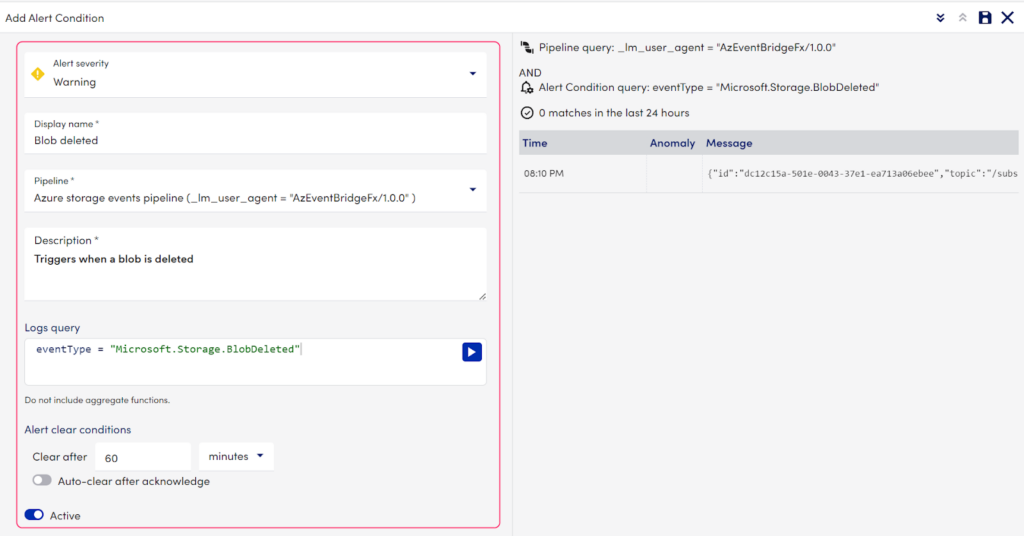
You can now test the alert rule by deleting a blob from your Azure Storage account. Upon deleting a blob from any container in the storage account, you should receive an alert notification in LogicMonitor, as shown in the following screenshot:
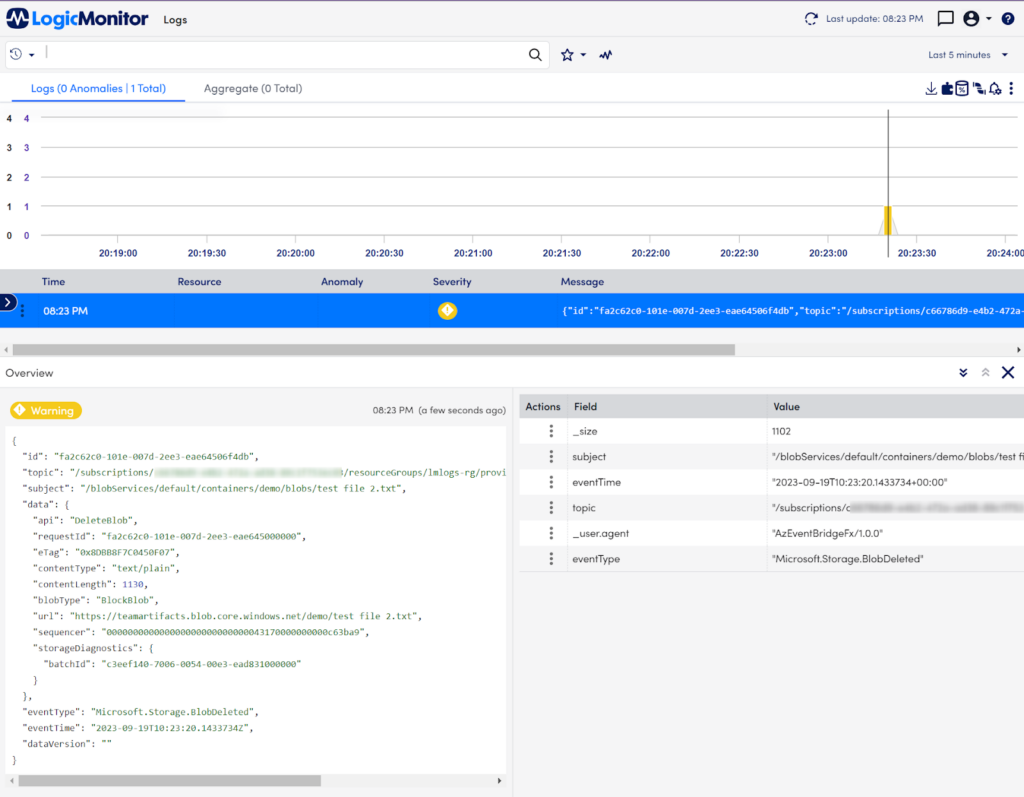
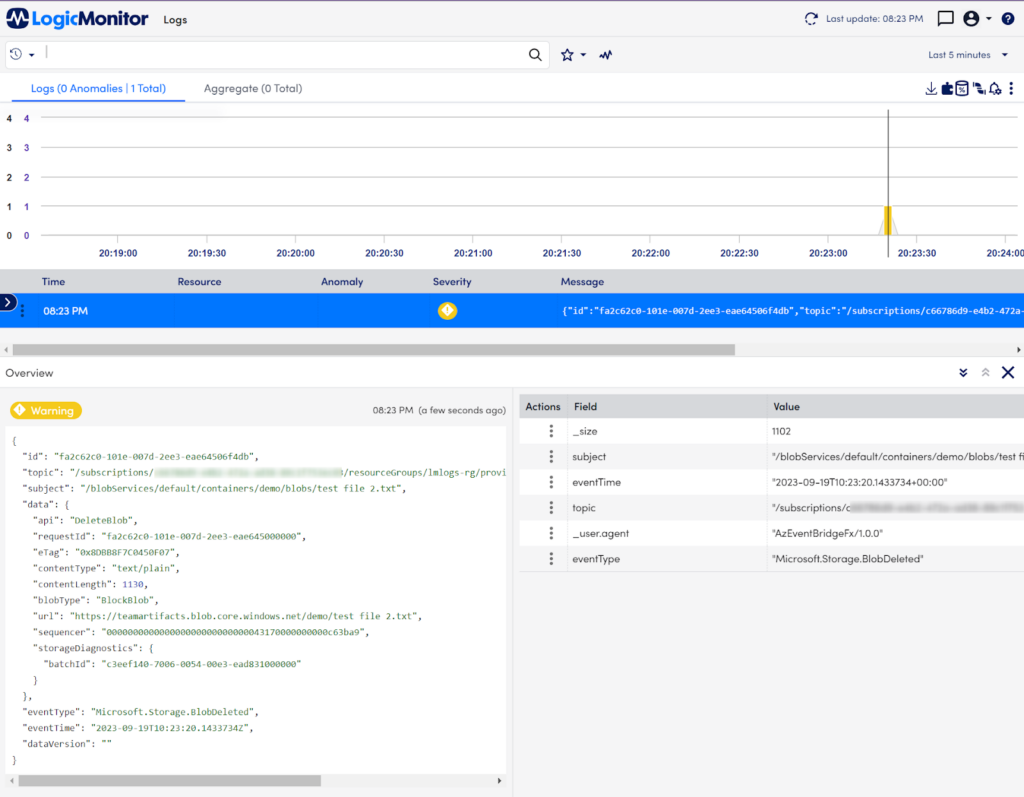
Likewise, you can create additional Event Grid subscriptions to forward events from other Azure resources to the AzureEventBridge function and set up alert rules in LogicMonitor logs to notify users of critical events. Here are some examples:
- Container Image Deletion Alert: Set up notifications for the removal of a container image from Azure Container Registry.
- Virtual Machine Configuration Alert: Ensure that any configuration changes to virtual machines trigger an alert, maintaining regulatory compliance.
- Azure Kubernetes Service Alert: Notify administrators of any changes to Azure Kubernetes Service to ensure that the cluster is always up-to-date.
Conclusion
Integrating Azure resources with LogicMonitor Logs provides a comprehensive view of your infrastructure’s health and activities. By leveraging Azure Event Grid and Azure Functions, you can seamlessly forward events to LogicMonitor and set up alerts for critical incidents. This integration ensures that you’re always informed about significant changes and can take timely action when necessary. To get started with LogicMonitor, please schedule a demo via our friendly chatbot or sign up for a free trial.