Executing Internal Web Checks using Groovy Scripts
Last updated - 19 October, 2023
You can also execute your Internal Web Check using a Groovy script to collect and process HTTP data. Internal Web Checks with at least a single step that runs using Groovy script consist of a Step Request script and a Step Response processor. Step Request can send a GET request to collect a site’s authenticating token and share the token while the Step Response authenticates into the site.
The following are some of the benefits of executing Internal Web Checks using Groovy scripts:
- More flexible and particularly useful for sites that use form-based authentication with dynamic tokens.
- Unique due to their ability to share contexts (dynamic tokens for authentication) between steps.
You can use the Groovy script while adding or editing your Internal Web Check. The following details the steps to create an Internal Web Check using Groovy script:
- In LogicMonitor, navigate to Websites > (Add) +> select Internal Web Check.
- At the Basic step of the wizard, enter the required details.
- Select Next:Settings.
- At the Setting step of the wizard, do the following:
- In the Default root URL section, select http:// or https:// protocol depending on your web server settings, and enter the website domain to which your Web Check request will be sent.
- In the Step One URL Path field, enter the first path that should be tested for your website. Enter the path relative to the website domain (For example,
/folder/page.htm
). - Select the Script tab under the Request section.
- Toggle on the Use Groovy Script for Request switch.
- Select Generate Script from Settings to have LogicMonitor auto-generate request scripts based on those settings.
- Select the Script tab under the Response section.
- Toggle on the Use Groovy Script for Response switch.
- Select Generate Script from Settings to have LogicMonitor auto-generate response scripts based on those settings.
- Select Next:Checkpoints.
- At the Checkpoints step of the wizard, specify the Collectors that will send the checks.
The Use Default Website Settings toggle is selected by default.
Note: Your user account must have Collector view permissions to view and select Collectors from this area of the dialog. For more information, see Roles. - Select Next: Alert Triggering.
- At the Alert Triggering step of the wizard, enter the required details.
- Select Finish.
Writing a Request Script
The request script uses the Groovy API to get an HTTP response.
API Commands for a Request Groovy Script
The following table lists the API commands you may use in your request Groovy script.
API | Return type | Description | Default value (if applicable) |
setAuthType(AuthType authType) | LMRequest | Set the Authentication Type | |
setUsername(String username) | LMRequest | Set the Authentication username | |
setPassword(String password) | LMRequest | Set the Authentication password | |
setDomain(String domain) | LMRequest | Set the Authentication Domain (only for NTLM Authentication) | |
followRedirect(boolean) | LMRequest | Specifies whether or not redirects should be followed | True |
addHeader(String name, String value) | LMRequest | Add the HTTP headers | |
userHttp1_0() | LMRequest | Specifies if HTTP/1.0 protocol version is used | False |
userHttp1_1() | LMRequest | Specifies if HTTP/1.1 protocol version is used | True |
needFullpageLoad() | LMRequest | Specifies if the full page needs to be loaded | False |
setContext(String name, Object value) | LMHttpClient | Set the context for the next scripts | |
getContext(String name) | Object | Get the context of the previous scripts | |
request(LMRequest) | LMHttpClient | Set the HTTP request parameters | |
get() | StatusCode | GET the Request and Return status. | |
get(URL) | StatusCode | GET the Request and Return status. | |
head() | StatusCode | Check if the URL exists | |
post(URL, PostDataType, String PostData) | StatusCode | Post the HTTP data | |
setProxy(String hostname, int port, String schema) | LMRequest | Set the HTTP proxy. Schema can only be HTTP or HTTPS | |
setProxy(String hostname, int port, String schema, String proxyUsername, String proxyPassword) | LMRequest | Set the HTTP proxy along with credentials. |
Example Request Script Commands
- The following Groovy syntax would be used in the first step of an Internal Web Check for a site using basic authentication.
import com.logicmonitor.service.groovyapi.AuthType; import com.logicmonitor.service.groovyapi.LMRequest; LMRequest request = new LMRequest(); request.setAuthType(AuthType.BASIC) .setUsername("username") .setPassword("password") .followRedirect(false) .useHttp1_0() .needFullpageLoad(true); String jsonData = "{\"name\": \"value\"}" return LMHttpClient.request(request) .post(JSON, jsonData);
- Example setContext() response:
StatusCode status = LMResponse.statusMatch(200); LMResponse.setContext("body", Response.getBody()); return status;
- Example getContext() response:
return LMResponse.plainTextmatch(Response.getContext("exampletext"))
- Example post(PostDataType, String PostData) response:
import static com.logicmonitor.service.groovyapi.StatusCode.*; import static com.logicmonitor.service.groovyapi.PostDataType.*; String jsonData = "{\"name\": \"value\"}"; return LMHttpClient.post(JSON, jsonData);
- Example request(LMRequest) response:
import com.logicmonitor.service.groovyapi.AuthType; import com.logicmonitor.service.groovyapi.LMRequest; LMRequest request = new LMRequest(); request.setAuthType(AuthType.BASIC) .setUsername("username") .setPassword("password") .followRedirect(false) .useHttp1_0() .needLoadFullPage(false); String jsonData = "{\"name\": \"value\"}" return LMHttpClient.request(request) .post(JSON, jsonData);
Writing a Response Script
The response script will parse the HTTP response from your request script and apply any post-processing methods (For example, check status, check the HTTP response body, and so on).
The following table lists the API calls you may use in your request Groovy script.
API Command | Return type | Description |
getStatus() | Integer | Return the HTTP status code |
getReasonPhase() | String | Return the HTTP Reason Phase |
getProtocolVersion() | String | Return the HTTP Protocol Version |
getHeaders() | Map (String, List [String]) | Return all the headers |
getHeader(String name) | List | Return the header value with name. If the header does not exist, a “null” response will be returned. Note that the name is case sensitive |
getBody() | String | Return the HTTP Response Body |
statusMatch(int expected) | StatusCode | Check if the status is as expected. If so, this will return STATUS_OK. Otherwise it will return STATUS_STATUS_MISMATCH |
regexMatch(String pattern) | StatusCode | Check if the regex pattern matches the HTTP Response Body. If it fails, return STATUS_CONTENTS_MISMATCH |
globMatch(String pattern) | StatusCode | Check if the regex pattern matches the HTTP Response Body. If it fails, return STATUS_CONTENTS_MISMATCH |
plainTextMatch(String pattern) | StatusCode | Check if the HTTP Response Body contains the plain text. If it fails, return STATUS_CONTENTS_MISMATCH |
jsonMatch(String path, String expectValue) | StatusCode | Check if the path’s JSON result matches the expect value. If it fails, return STATUS_CONTENTS_MISMATCH |
PathMatch(String path, String expectValue) | StatusCode | Check if the string’s path matches the expect value. If it fails, return STATUS_CONTENTS_MISMATCH |
keyValueMatch(String key, String expectValue) | StatusCode | Check if the returned key/Values match the expect value. If it fails, return STATUS_CONTENTS_MISMATCH |
setContext(String name, Object value) | void | Sets the context for the next stages of your script |
getContext(String) | Object | Return the context set in a previous script stage |
Example Response Script
In the event that you wanted to verify a response status of “302” for a site, use the following request:
import com.logicmonitor.service.groovyapi.LMRequest;
LMRequest request = new LMRequest();
return LMHttpClient.request(request.followRedirect(false)
.get());
You would write your response script as follows:
return LMResponse.statusMatch(302);
Status Codes
Code | Value | Description |
STATUS_OK | 1 | Data collection functioned as expected |
STATUS_MISMATCH | 11 | The HTTP response status does not match |
STATUS_CONTENTS_MISMATCH | 12 | The HTTP Request did not return expected HTTP Response body |
Full Example Script
Below is a full two-step script used for verifying the availability of a messaging service. This particular script uses a dynamic token shared between steps one and two of the Internal Web Check through the setContext and getContext commands in order to authenticate into the site. The post-processing method looks for the presence of “Welcome” in the HTTP response to verify the site’s availability.
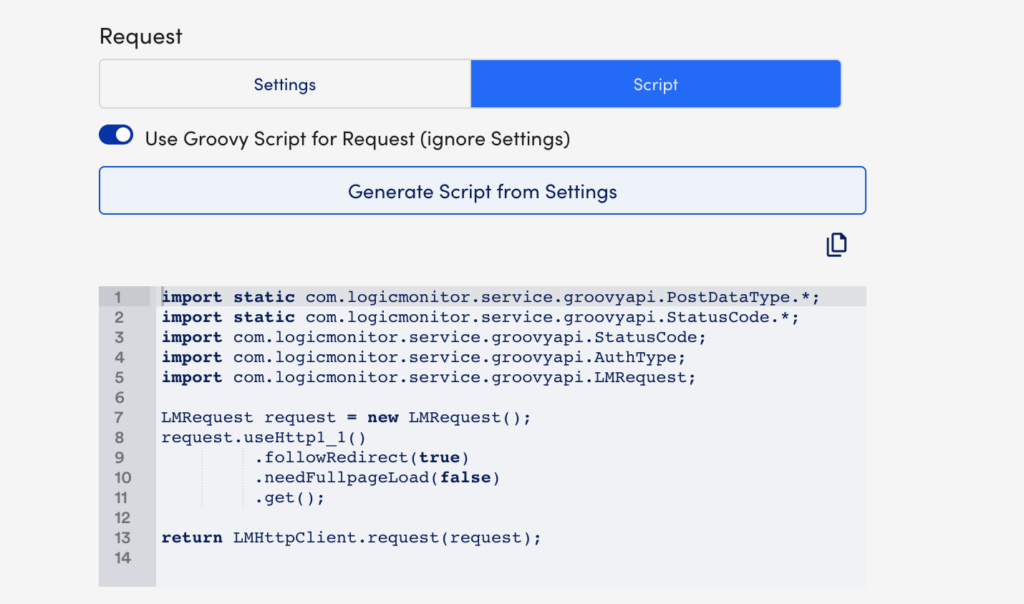
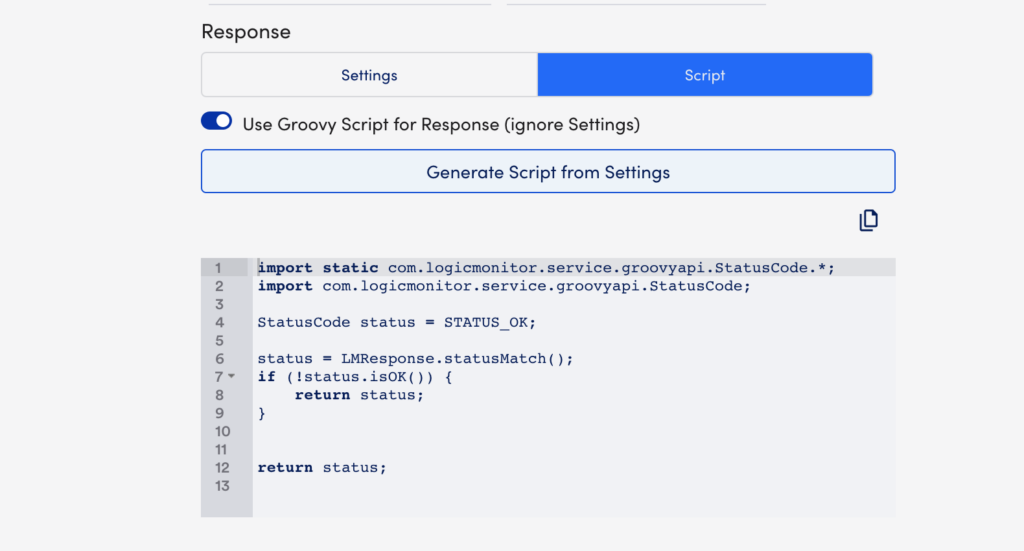
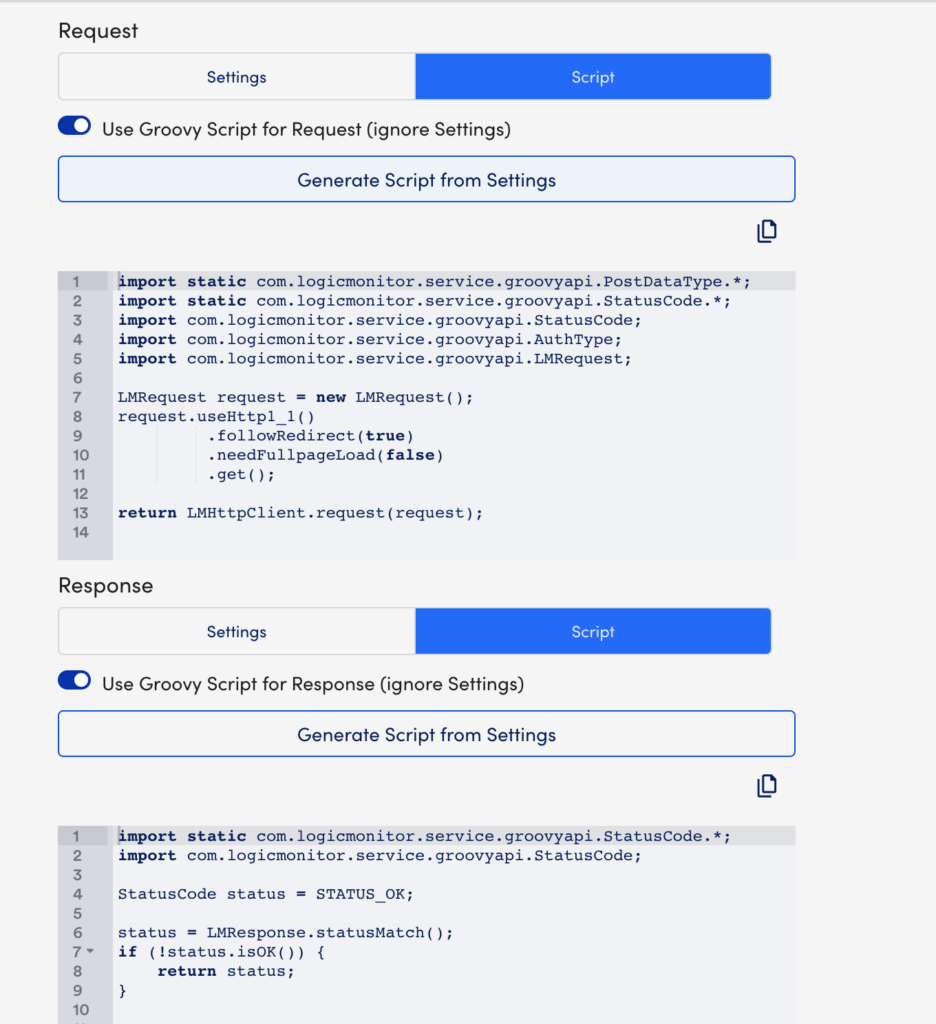